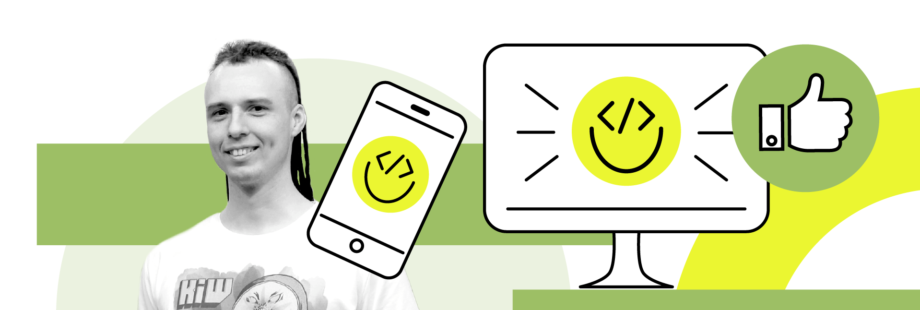
What is the Optimistic UI?
Konrad JaguszewskiReading Time: 4 minutes
Optimistic UI can be confusing. Many people see web performance as something that is strictly technical. Engineers push servers to the limit. Developers do all they can to build APIs that return responses in a blink of an eye. They optimize images and use the latest technologies. Often it’s still not enough, because users don’t care how much work you put into the server infrastructure or how much you compressed the images. At the end of the day, performance is not only about technology, but about how smooth the user experience is.
That’s where the Optimistic UI comes in. It makes your app run faster. Or at least that’s how users will see it. Optimistic UI is a magic trick and like a true magic trick - it requires a little bit of cheating.
Being optimistic means being hopeful and confident about the future. Essentially, the Optimistic UI comes exactly to that. To explain this, let’s look into how the traditional UI works. To keep it simple, we will focus on the well-known “Like” button. However, Optimistic UI can be used in many different cases (like messenger applications or adding items to a cart) and probably your product could use it too.
The old way (without Optimistic UI)
If you remember websites with guest books, visitors counters and a lot of flashy GIFs, there is a good chance that you are familiar with this pattern:
- You click a button.
- API request is sent.
- You wait for the response.
- The page is updated to reflect the changes.
It looks like this:
We quickly realized that it’s not a very good user experience. The problem is that there is no indication that something is happening in the background. Users don’t like to wait and even more they don’t like when there is no reaction to their action. So we came up with a solution to make their journey a little smoother.
The better way (still no Optimistic UI)
We, developers, wanted to let users know that after they clicked a button, we started working on their request. Something is happening in the background and we just ask for a little patience.
This is how we improved it:
- You click a button.
- Button goes into a loading state.
- API request is sent.
- You wait for the response (but at least you know that something is happening).
- The page is updated to reflect the changes.
Now users see immediate reaction to their mouse click. There is a nice loading icon, so they know that while they’re waiting we are processing their request. They should be happy, right? Well, not really, because they still don’t want to wait.
The optimistic way (with Optimistic UI)
According to the RAIL model, response to users actions between 0 and 100 ms feels immediate to them. Response longer than that breaks the connection between action and reaction. And according to this survey, the average server response time is 538 ms (data from October 2021). This shows two things - we are impatient and the servers are not there yet to solve this problem. This is where Optimistic UI comes to the rescue!
Let’s look at this pattern now:
- You click a button.
- The page is updated immediately.
Seems like magic? Seems like cheating? That’s because it is (just a bit). We are updating the UI, because we are confident about the future.
The fact is that an API should return a successful response 99% of the time. In the example of the “Like” button, the API should only return an error when there is a problem with the network or the server. In that case, we can simply show to the users that something went wrong or just revert the changes, but the great majority of them will not encounter this.
When using the Optimistic UI approach, we are changing the page immediately without waiting for the response from the server. The request is still being processed in the background, but the user doesn’t have to know about this.
Fun fact: On Facebook, the request behind “Like” takes around 1.5 seconds. Imagine waiting that long if it wasn’t implementing the Optimistic UI. In 1.5 seconds I’ve already scrolled through 3 posts!
Where do we go from here?
A user that doesn’t have to wait is a happy user. With the Optimistic UI, users don’t get irritated and the chances of them leaving a website are decreasing. It seems like we’ve reached peak performance. But wait, there is more!
Modern JavaScript frameworks and tools brought a lot of improvements. From the developer’s point of view, they made implementing the Optimistic UI much smoother. React and Apollo are among the most widely used tools, so let’s check how they can make our life easier when it comes to the Optimistic UI.
With Apollo we can utilize an optimisticResponse
option in the mutate
function. Thanks to that, we can show an expected response immediately and then update the UI with the actual value (which should be the same).
Here’s a little example from the Apollo documentation:
// Mutation definition
const UPDATE_COMMENT = gql`
mutation UpdateComment($commentId: ID!, $commentContent: String!) {
updateComment(commentId: $commentId, content: $commentContent) {
id
__typename
content
}
}
`;
// Component definition
function CommentPageWithData() {
const [mutate] = useMutation(UPDATE_COMMENT);
return (
<Comment
updateComment={({ commentId, commentContent }) =>
mutate({
variables: { commentId, commentContent },
optimisticResponse: {
updateComment: {
id: commentId,
__typename: "Comment",
content: commentContent
}
}
})
}
/>
);
}
As you can see, implementing the Optimistic UI doesn’t have to be a tedious task.
Final words
Optimistic UI is a great improvement to the user experience. The “Like” button is not the only situation where it can be applied. It actually can be used in many places where API responses are predictable. Maybe even while reading this post, you came up with ideas where this approach could help your product.